Differences between React Element and Component
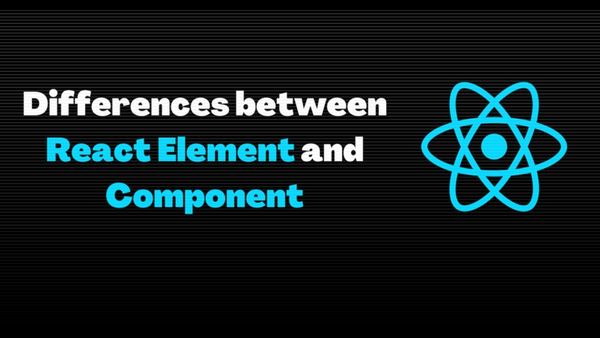
In this Article we are going to discuss about Differences between React Element, Component and how to create an element, How to update element, and How to create class and Function elements.
The building units of the apps you create using ReactJS are elements and components. Understandably, these are the most fundamental ideas you need to learn before working with React. Although both elements and components serve as structural or functional parts of your code, they operate substantially different from one another. Let us examine how.
One of the most prominent libraries used by developers today to create flexible, responsive UIs for applications is ReactJS. The Facebook-hosted library is a JavaScript library with several unique characteristics that make it stand out from competing frameworks. ReactJS technology is committed to making the process of creating apps simpler for us, but it also makes sure that the final products created with it run at their peak. Applications made using ReactJS are quite immersive thanks to features like virtual DOM. Other characteristics, including reusable components, ease the process of developing apps for us. Because of the time and resource savings, we can use our imagination to design React apps. You are likely to understand the fundamental ideas of ReactJS if you are new to React or have chosen to learn it because of these features. The first of these concepts are elements and components in ReactJS.
Are elements and components equivalent?
Not at all, no. One may argue that the most crucial concept to comprehend or learn about in ReactJS is the concept of components. Both JavaScript and React’s elements could be compared in this way. In ReactJS, elements serve as the building blocks of your code, while components put your user interface together. Props are used as input by components to create element trees as returns. They combine to form the core foundation of your ReactJS-based UI. Because of this, one of the first things you learn when learning ReactJS or JavaScript is the idea of elements and components. You could initially think of the two as being somewhat comparable because they are both significant aspects of your code. They receive data inputs and output actions to the user interface. Only a tiny deeper inspection would reveal that they are two quite different categories of ReactJS components.
In the simplest terms possible, “React apps’ smallest building parts are called elements.” React uses simple Javascript objects for its elements, which are not to be mistaken with browser DOM elements.
What are Elements?
A JavaScript concept is objects. Simply said, objects are just things with unique types and characteristics. Similar to how a pen has specific characteristics like color, shape, and varieties like ball pens or fountain pens, JS objects too have assigned characteristics that specify their characters. Examples of objects in JS include the display of a welcome message, the timestamp, and others. Apart from primitives (such as the value of pi), everything in Javascript is an object. They often consist of a mix of data structures, functions, and variables. Objects are a set of name values. They make our codes easier to read by becoming a compilation.
How to create an element in ReactJS?
The best way to describe creating an element in React Elements is to state what we want to see on the screen. Real DOMs are handled by React virtual DOMs to match React elements. Components are made up of elements. In the part after this, we shall talk about it. Let’s look at an example of creating an element in React.
React.createElement() was used to generate the element below. It is a function that considers the element’s type, properties, and children. In this case, we constructed an element with h1 parameters that have null properties. As its children, the string “Hello Reader” is passed.
import React from ‘react’;
import React from ‘react’;
import ReactDOM from ‘react-dom’;
// Without JSX
const ele1 = React.createElement(
‘h1’,
{id:’header’},
‘Hello Learner’
);
ReactDOM.render(ele1,document.getElementById(‘root’));
This would return the following object:
{
type: ‘h1’,
props: {
children: ‘Hello Learner’,
id: ‘header’
}
}
How to update elements in ReactJS?
Once built, React elements cannot have their children or attributes modified. Here’s an analogy to think about. A single frame in a video is an example of an element. Frames in the video can be added, deleted, or exchanged to create modifications. The only way to update elements is to replace them with new ones. The element is passed through root.render() to do this. ReactJS makes this relatively simple because its elements are both cheap to produce and easy to use. As I previously stated, the virtual DOM compares an element’s children and attributes and only makes the necessary DOM adjustments to get it to the desired state. As the entire UI does not need to be refreshed, this helps to reduce bugs. In essence, updating a component just requires changing one element, or one line of code throughout the entire component.
Let’s look at an element that shows the time as an example. This component has to be updated every second to function properly. If elements are immutable, how can this be accomplished? The element is updated in the example below as every second, a setInterval() callback calls root.render()
const root = ReactDOM.createRoot(
document.getElementById(‘root’)
);
function tick() {
const element = (
<div>
<h1>Hello Learner </h1>
<h2>It is {new Date().toLocaleTimeString()}.</h2>
</div>
);
root.render(element);
}
setInterval(tick, 1000);
What are Components?
The UI of React apps is made up of components. They serve as the UI’s blueprints or templates. ReactJS enables us to divide the user interface into components, which is a better way to put it. Individual UI elements can be controlled separately in this way. Components function as separate, reusable parts.
Components are functions in Javascript, so to speak. To specify what should be shown on the screen, they return react elements, which accept arbitrary inputs known as props. ReactJS has two different sorts of components: class components and function components.
How to create function components in ReactJS?
Simple js functions form function components. They accept data-only arguments for solitary props and produce the output of a react element. Prop stands for properties. Let’s look at an illustration of a function component with a passing user as the prop.
import React from ‘react’;
import ReactDOM from ‘react-dom’;
function Welcome(user){
return <div>
<h3> Hello {user.name}</h3>
</div>
}
const ele = <Welcome name=”Learner”/>
ReactDOM.render(ele,document.getElementById(“root”));
How to create a class component in ReactJS?
The ES6 class is used to declare a component in class components. Components can be divided into smaller components, and smaller components can be made up of larger components. This makes it easier to remove and reuse component parts that might otherwise be challenging. This might be because complicated components frequently include a considerable amount of nesting. Props cannot be changed. There are two types of functions: pure and impure. Props are constant in pure functions but variable in impure functions. All components in React must treat props as though they are pure functions, as per a stringent rule of thumb in React. State can be used to update dynamic props. State allows components to update their props and store variables in a single source. Let’s use state variables to construct a ticking clock class component.
class App extends React.Component {
state = {
date: “”
};
getDate() {
var date = { currentTime: new Date().toLocaleString() };
this.setState({
date: date
});
}
render() {
return (
<div class=”date”>
<p> ddd {this.state.date}</p>
</div>
);
}
}
export default App;
The distinctions between an element and a component in ReactJS
We now clearly understand that the characters and uses of ReactJS’s elements and components are entirely different. UIs in React is created using components, which are created using elements.
Let’s quickly review the main distinctions between React elements and components.
Elements | Components |
Components are always required to return elements. | Components may choose to accept input to return elements. |
Elements are objects which do not have methods. | There are life cycle methods for every component. |
Individual nodes in the DOM tree are represented by elements. | Components comprise a DOM tree. |
Elements are immutable. | Mutable states can exist in components. |
Elements cannot be utilized with React hooks since they are immutable. | Functional components can employ React hooks. |
Elements are lighter as they lack states. | Components are larger as they often have states. |
Elements render more quickly and are easier to construct. | Components are more complicated and thus render slower than elements |
- Machine Learning : An Introductory Review Of Machine Learning
- Artificial Intelligence: A Powerful Tool of Future
- Is Artificial Intelligence a Threat or a Benefit?
To sum up
This topic about the distinctions between elements and components in ReactJS has come to an end. There is a hierarchical distinction between elements and components even if both serve as building pieces. Components created from elements result in the user interfaces (UIs) of React apps. As a result, there are some noticeable variances in terms of the creation process and material makeup of elements and components. To make them easier to understand, we have summarized them in the table above. The fundamental building block for building ReactJS applications is learning how to create elements and components. I hope I was able to dispel any confusion you may have had on the differences between the two.